...
for i in range(33,126+1):
print("i={0:3d}-->{1:1s} ".format(i,chr(i)),end="\t")
if (i-32)%7==0:print()
i= 33-->! i= 34-->" i= 35--># i= 36-->$ i= 37-->% i= 38-->& i= 39-->'
i= 40-->( i= 41-->) i= 42-->* i= 43-->+ i= 44-->, i= 45-->- i= 46-->.
i= 47-->/ i= 48-->0 i= 49-->1 i= 50-->2 i= 51-->3 i= 52-->4 i= 53-->5
i= 54-->6 i= 55-->7 i= 56-->8 i= 57-->9 i= 58-->: i= 59-->; i= 60--><
i= 61-->= i= 62-->> i= 63-->? i= 64-->@ i= 65-->A i= 66-->B i= 67-->C
i= 68-->D i= 69-->E i= 70-->F i= 71-->G i= 72-->H i= 73-->I i= 74-->J
i= 75-->K i= 76-->L i= 77-->M i= 78-->N i= 79-->O i= 80-->P i= 81-->Q
i= 82-->R i= 83-->S i= 84-->T i= 85-->U i= 86-->V i= 87-->W i= 88-->X
i= 89-->Y i= 90-->Z i= 91-->[ i= 92-->\ i= 93-->] i= 94-->^ i= 95-->_
i= 96-->` i= 97-->a i= 98-->b i= 99-->c i=100-->d i=101-->e i=102-->f
i=103-->g i=104-->h i=105-->i i=106-->j i=107-->k i=108-->l i=109-->m
i=110-->n i=111-->o i=112-->p i=113-->q i=114-->r i=115-->s i=116-->t
i=117-->u i=118-->v i=119-->w i=120-->x i=121-->y i=122-->z i=123-->{
i=124-->| i=125-->} i=126-->~
------------------------------------------------------help()
>>> help()
Welcome to Python 3.9's help utility!
...
To quit this help utility and return to the interpreter, just type "quit".
...
-----------------------------------pyhton 關鍵字
help> keywords
Here is a list of the Python keywords. Enter any keyword to get more help.
False break for not
None class from or
True continue global pass
__peg_parser__ def if raise
and del import return
as elif in try
assert else is while
async except lambda with
await finally nonlocal yield
-----------------------------------pyhton 模組
help> modules
Please wait a moment while I gather a list of all available modules...
__future__ argparse help sched
__main__ array help_about scrolledlist
_abc ast history search
_aix_support asynchat hmac searchbase
_ast asyncio html searchengine
_asyncio asyncore http secrets
_bisect atexit hyperparser select
_blake2 audioop idle selectors
_bootlocale autocomplete idle_test setuptools
_bootsubprocess autocomplete_w idlelib shelve
_bz2 autoexpand imaplib shlex
_codecs autopep8 imghdr shutil
_codecs_cn base64 imp sidebar
_codecs_hk bdb importlib signal
_codecs_iso2022 binascii inspect site
_codecs_jp binhex io smtpd
_codecs_kr bisect iomenu smtplib
_codecs_tw browser ipaddress sndhdr
_collections builtins itertools socket
_collections_abc bz2 json socketserver
_compat_pickle cProfile keyword sqlite3
_compression calendar lib2to3 squeezer
_contextvars calltip linecache sre_compile
_csv calltip_w locale sre_constants
_ctypes cgi logging sre_parse
_ctypes_test cgitb lzma ssl
_datetime chunk macosx stackviewer
_decimal cmath mailbox stat
_distutils_hack cmd mailcap statistics
_elementtree code mainmenu statusbar
_functools codecontext marshal string
_hashlib codecs math stringprep
_heapq codeop mimetypes struct
_imp collections mmap subprocess
_io colorizer modulefinder sunau
_json colorsys msilib symbol
_locale compileall msvcrt symtable
_lsprof concurrent multicall sys
_lzma config multiprocessing sysconfig
_markupbase config_key netrc tabnanny
_md5 configdialog nntplib tarfile
_msi configparser nt telnetlib
_multibytecodec contextlib ntpath tempfile
_multiprocessing contextvars nturl2path test
_opcode copy numbers textview
_operator copyreg opcode textwrap
_osx_support crypt operator this
_overlapped csv optparse threading
_peg_parser ctypes os time
_pickle curses outwin timeit
_py_abc dataclasses parenmatch tkinter
_pydecimal datetime parser token
_pyio dbm pathbrowser tokenize
_queue debugger pathlib toml
_random debugger_r pdb tooltip
_sha1 debugobj percolator trace
_sha256 debugobj_r pickle traceback
_sha3 decimal pickletools tracemalloc
_sha512 delegator pip tree
_signal difflib pipes tty
_sitebuiltins dis pkg_resources turtle
_socket distutils pkgutil turtledemo
_sqlite3 doctest platform types
_sre dynoption plistlib typing
_ssl editor poplib undo
_stat email posixpath unicodedata
_statistics encodings pprint unittest
_string ensurepip profile urllib
_strptime enum pstats uu
_struct errno pty uuid
_symtable faulthandler py_compile venv
_testbuffer filecmp pyclbr warnings
_testcapi fileinput pycodestyle wave
_testconsole filelist pydoc weakref
_testimportmultiple fnmatch pydoc_data webbrowser
_testinternalcapi format pyexpat window
_testmultiphase formatter pyparse winreg
_thread fractions pyshell winsound
_threading_local ftplib query wsgiref
_tkinter functools queue xdrlib
_tracemalloc gc quopri xml
_uuid genericpath random xmlrpc
_warnings getopt re xxsubtype
_weakref getpass redirector zipapp
_weakrefset gettext replace zipfile
_winapi glob reprlib zipimport
_xxsubinterpreters graphlib rlcompleter zlib
_zoneinfo grep rpc zoneinfo
abc gzip run zoomheight
aifc hashlib runpy zzdummy
antigravity heapq runscript
---------------------
help> math
Help on built-in module math:
NAME
math
DESCRIPTION
This module provides access to the mathematical functions
defined by the C standard.
FUNCTIONS
acos(x, /)
Return the arc cosine (measured in radians) of x.
The result is between 0 and pi.
...
---------------------
help> modules math
Here is a list of modules whose name or summary contains 'math'.
If there are any, enter a module name to get more help.
cmath - This module provides access to mathematical functions for complex
math - This module provides access to the mathematical functions
math_test
math
test.test_cmath
test.test_math
PIL.ImageMath
PIL._imagingmath
django.db.models.functions.math
numpy.core._multiarray_umath
numpy.core._umath_tests
numpy.core.tests.test_scalarmath
numpy.core.tests.test_umath
numpy.core.tests.test_umath_accuracy
numpy.core.tests.test_umath_complex
numpy.core.umath - Create the numpy.core.umath namespace for backward compatibility. In v1.16
numpy.core.umath_tests - Shim for _umath_tests to allow a deprecation period for the new name.
numpy.lib.scimath - Wrapper functions to more user-friendly calling of certain math functions
numpy.linalg._umath_linalg
pygame.math
pygame.tests.math_test
import math
dir()
['__annotations__', '__builtins__', '__doc__', '__loader__', '__name__', '__package__', '__spec__', 'math']
dir(math)
['__doc__', '__loader__', '__name__', '__package__', '__spec__', 'acos', 'acosh', 'asin', 'asinh', 'atan', 'atan2', 'atanh', 'cbrt', 'ceil', 'comb', 'copysign', 'cos', 'cosh', 'degrees', 'dist', 'e', 'erf', 'erfc', 'exp', 'exp2', 'expm1', 'fabs', 'factorial', 'floor', 'fmod', 'frexp', 'fsum', 'gamma', 'gcd', 'hypot', 'inf', 'isclose', 'isfinite', 'isinf', 'isnan', 'isqrt', 'lcm', 'ldexp', 'lgamma', 'log', 'log10', 'log1p', 'log2', 'modf', 'nan', 'nextafter', 'perm', 'pi', 'pow', 'prod', 'radians', 'remainder', 'sin', 'sinh', 'sqrt', 'tan', 'tanh', 'tau', 'trunc', 'ulp']
help(math.pow)
Help on built-in function pow in module math:
pow(x, y, /)
Return x**y (x to the power of y).
help> quit
You are now leaving help and returning to the Python interpreter.
If you want to ask for help on a particular object directly from the
interpreter, you can type "help(object)". Executing "help('string')"
has the same effect as typing a particular string at the help> prompt.
>>>
------------------------------------------------------
>>> import this
The Zen of Python, by Tim Peters python 禪學
進制轉換
*******************************************
chr(65)
'A'
ord("A")
65
bin(65)
'0b1000001'
oct(65)
'0o101'
hex(65)
'0x41'
int(0b1000001)
65
int(0o101)
65
int(0x41)
65
"{0:b}".format(65)
'1000001'
"{0:o}".format(65)
'101'
"{0:x}".format(65)
'41'
"{0:d}".format(0b1000001)
'65'
"{0:d}".format(0o101)
'65'
"{0:d}".format(0x41)
'65'
format(65,"b")
'1000001'
format(65,"o")
'101'
format(65,"x")
'41'
format(0b1000001,"d")
'65'
format(0o101,"d")
'65'
format(0x41,"d")
'65'
print("%b" %(65))
Traceback (most recent call last):
File "<pyshell#39>", line 1, in <module>
print("%b" %(65))
ValueError: unsupported format character 'b' (0x62) at index 1
print("%o" %(65))
101
print("%x" %(65))
41
--------------------------------------i、d 皆代表10進制
print("%d" %(0b1000001))
65
print("%d" %(0o101))
65
print("%d" %(0x41))
65
print(f'{65:b}')
1000001
print(f'{65:o}')
101
print(f'{65:x}')
41
----------------------------10進制轉2、8、16----------------------------
bin(65)
'0b1000001'
format(65,"b")
'1000001'
#沒此用法
print("%b" %65)
Traceback (most recent call last):
File "<pyshell#59>", line 1, in <module>
print("%b" %65)
ValueError: unsupported format character 'b' (0x62) at index 1
oct(65)
'0o101'
format(65,"o")
'101'
print("%o" %65)
101
hex(65)
'0x41'
format(65,"x")
'41'
print("%x" %65)
41
----------------------------2、8、16進制轉10----------------------------
int(0b1000001)
65
int("0b1000001",2)
65
int("1000001",2)
65
int(0o101)
65
int("0o101",8)
65
int("101",8)
65
int(0x41)
65
int("0x41",16)
65
int("41",16)
65
----------------------------16進制轉2---------------------------
bin(0x41)
'0b1000001
----------------------------2進制轉16---------------------------
hex(0b1000001)
'0x41'
------------------------------------------------------10進制小數點轉2進制
def ConvertDecimalFractionToBinary(Fraction):
x=Fraction
RtnStr=""
while x>0:
x=x*2
if x>=1:
RtnStr=RtnStr+"1"
x=x-1
else:
RtnStr=RtnStr+"0"
return (RtnStr)
Franction=float(input("輸入任正小數:"))
print(str(Franction)+"轉成二進制="+ConvertDecimalFractionToBinary(Franction))
=======
輸入任正小數:0.8125
0.8125轉成二進制=1101
def DecToBin(x):
LstBin=[]
while x:
x *=2
LstBin.append(1 if x>=1 else 0 )
x -= int(x)
return LstBin
n=0.3
print(DecToBin(n))
=========
[0, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1]
+++++++
def DecToBin(x):
LstBin=[]
while x:
x *=2
LstBin.append("1" if x>=1 else "0" )
x -= int(x)
#print(LstBin)
strBin="0."+"".join(LstBin)
return strBin
n=0.1
print(DecToBin(n))
=========
(0.1)=0.0001100110011001100110011001100110011001100110011001101 (0011循环)
(0.2)=0.001100110011001100110011001100110011001100110011001101 (0011循环)
(0.3)=0.010011001100110011001100110011001100110011001100110011 (1001循环)
(0.4)=0.01100110011001100110011001100110011001100110011001101 (0110循环)
++++++
def dec2bin(x):
x -= int(x)
bins = []
while x:
x *= 2
bins.append(1 if x>=1. else 0)
x -= int(x)
return bins
#print(dec2bin(.8125))
# [1, 1, 0, 1]
print(dec2bin(0.1))
def bin2dec(b):
d = 0
for i, x in enumerate(b):
d += 2**(-i-1)*x
return d
#print(dec2bin(0.8125))
# [1, 1, 0, 1]
#print(bin2dec(dec2bin(0.8125)))
# 0.8125
print(bin2dec(dec2bin(0.1)))
#print(bin2dec([1, 1, 0, 1]))
++++
https://segmentfault.com/a/1190000020953696
------------------------------------------------------???
for i in range(0,10+1):
print("0.1+",(i/10),"=",0.1+(i/10))
=========
0.1+ 0.0 = 0.1
0.1+ 0.1 = 0.2
0.1+ 0.2 = 0.30000000000000004
0.1+ 0.3 = 0.4
0.1+ 0.4 = 0.5
0.1+ 0.5 = 0.6
0.1+ 0.6 = 0.7
0.1+ 0.7 = 0.7999999999999999
0.1+ 0.8 = 0.9
0.1+ 0.9 = 1.0
0.1+ 1.0 = 1.1
------------------------------
>>> format(65,'b')
'1000001'
>>> format(65,'o')
'101'
>>> format(65,'x')
'41'
char to ascii
*******************************************
chr(65)
'A'
ord('A')
65
----------------------------
>>> print('\101') #8進制
A
>>> print('\x41') #16進制
A
------------------------------------------------------使用標準涵式庫
>>> dir()
['__annotations__', '__builtins__', '__doc__', '__loader__', '__name__', '__package__', '__spec__']
>>> import math as MT
>>> dir()
['MT', '__annotations__', '__builtins__', '__doc__', '__loader__', '__name__', '__package__', '__spec__']
>>> dir(MT)
['__doc__', '__loader__', '__name__', '__package__', '__spec__', 'acos', 'acosh', 'asin', 'asinh', 'atan', 'atan2', 'atanh', 'ceil', 'comb', 'copysign', 'cos', 'cosh', 'degrees', 'dist', 'e', 'erf', 'erfc', 'exp', 'expm1', 'fabs', 'factorial', 'floor', 'fmod', 'frexp', 'fsum', 'gamma', 'gcd', 'hypot', 'inf', 'isclose', 'isfinite', 'isinf', 'isnan', 'isqrt', 'lcm', 'ldexp', 'lgamma', 'log', 'log10', 'log1p', 'log2', 'modf', 'nan', 'nextafter', 'perm', 'pi', 'pow', 'prod', 'radians', 'remainder', 'sin', 'sinh', 'sqrt', 'tan', 'tanh', 'tau', 'trunc', 'ulp']
>>> help(MT.ceil)
Help on built-in function ceil in module math:
ceil(x, /)
Return the ceiling of x as an Integral.
This is the smallest integer >= x.
>>> for i in range(LstMath.index('acos'),len(LstMath)):
print("{0:10s}".format(LstMath[i]),end='')
acos acosh asin asinh atan atan2 atanh ceil comb copysign cos cosh degrees dist e erf erfc exp expm1 fabs factorial floor fmod frexp fsum gamma gcd hypot inf isclose isfinite isinf isnan isqrt lcm ldexp lgamma log log10 log1p log2 modf nan nextafter perm pi pow prod radians remainder sin sinh sqrt tan tanh tau trunc ulp
>>> del MT
>>> dir()
['__annotations__', '__builtins__', '__doc__', '__loader__', '__name__', '__package__', '__spec__']
弧度又叫弳度。單位弧度定義做圓弧長度(arc length)等於半徑(radius)時的圓心角。角度以弧度做單位時,通常不寫單位,有時記做rad(㎭)。一個完整圓,弧度係2π,所以2π rad = 360°
*******************************************
>>> str1="HelloDay!"
>>> type(str1)
<class 'str'>
>>> for i in range(len(str1)):
print("{0:2d}={1:1s}".format(i,str1[i]),end='')
0=H 1=e 2=l 3=l 4=o 5=D 6=a 7=y 8=!
[ ] 切片 Slicing 運算
--------------------------------
>>> str1[5] //index=5
'D'
>>> str1[5:] //index=5~最後
'Day!'
>>> str1[5:7] //index=5~7 即(6+1)
'Da'
>>> str1[:] //複製
'HelloDay!'
>>> str1[::-1] //反轉
'!yaDolleH'
>>> str1[2:7:2] //index=2~(6+1) 間格2
'loa'
>>> str1[6::4] //index=6~ 間格4,不足又從index=6 開始
'a'
字串常用函式
--------------------------------
>>> str1.find('o')
4
>>> str1.count('l')
2
>>> str2=str1.replace("Day"," World")
>>> str2
'Hello World!'
>>> str1
'HelloDay!' //原字串不變
>>> str2.split() //str~list
['Hello', 'World!']
>>> str2
'Hello World!' //原字串不變
>>> str3=["Python","is","fun"]
>>> ' '.join(str3) //list~str
'Python is fun'
>>> str3
['Python', 'is', 'fun'] //原串列不變
*******************************************
>>> dictStu={'小萌': '1001', '小智': '1002', '小强': '1003', '小明': '1004'}
>>> dictStu
{'小萌': '1001', '小智': '1002', '小强': '1003', '小明': '1004'}
>>> dictStu.keys()
dict_keys(['小萌', '小智', '小强', '小明'])
>>> dictStu.values()
dict_values(['1001', '1002', '1003', '1004'])
>>> dictStu.items()
dict_items([('小萌', '1001'), ('小智', '1002'), ('小强', '1003'), ('小明', '1004')])
key --> value
-------------------------
>>> list(dictStu.keys())
['小萌', '小智', '小强', '小明']
>>> list(dictStu.values())
['1001', '1002', '1003', '1004']
>>> list(dictStu.values()).index('1002')
1
value --> key
------------------------------
>>> list(dictStu.keys())[list(dictStu.values()).index('1002')]
'小智
>>> [k for k,v in dictStu.items() if v=='1002']
['小智']
反轉原dcit
>>> {v:k for k,v in dictStu.items()}['1002']
'小智'
list預設值
*******************************************
>>> Lst=[i for i in range(0,10+1) if i%2==0]
>>> Lst
[0, 2, 4, 6, 8, 10]
>>> sum(i for i in range(1,10+1))
55
>>> sum(i for i in range(1,10+1) if i%2==0)
30
>>> sum(i for i in range(1,10+1) if i%2!=0)
25
>>> Lst=[i for i in range(1,10+1)]
>>> print(Lst)
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
>>> Lst=[i for i in range(1,10+1) if i%2==0]
>>> print(Lst)
[2, 4, 6, 8, 10]
Divide And Conquer:
Recursive:
1. Divide
2. Conquer
3. Combine
*******************************************
from math import floor
Recsiv=0
def conquer(Lst,PointerLeft,PointerRight):
global Recsiv
Recsiv+=1
print("Recsiv=",Recsiv," Lst=",Lst)
if PointerLeft<PointerRight:
print("PointerLeft=",PointerLeft," PointerRight=",PointerRight)
PointerMid=floor((PointerRight+PointerLeft)/2)
print("PointerMid=",PointerMid)
print()
print("Recsiv=",Recsiv," 目前Lst=",Lst[PointerLeft:PointerMid])
print()
print("Enter Recursive Again!!")
conquer(Lst,PointerLeft,PointerMid)
print("Recsiv=",Recsiv," 回上層,進入遞迴前的堆疊,繼續往下執行,Lst=",Lst[PointerLeft:PointerMid])
#print(Lst[PointerMid+1:PointerRight])
#conquer(Lst,PointerMid+1,PointerRight)
#print(Lst[PointerMid+1:PointerRight])
return Lst
else:
print("已不符遞迴條件!!")
print()
data=[50,60,45,30,90,20,80,15]
print("\nFinal Result:",conquer(data,0,8))
==========
Recsiv= 1 Lst= [50, 60, 45, 30, 90, 20, 80, 15]
PointerLeft= 0 PointerRight= 8
PointerMid= 4
Recsiv= 1 目前Lst= [50, 60, 45, 30]
Enter Recursive Again!!
Recsiv= 2 Lst= [50, 60, 45, 30, 90, 20, 80, 15]
PointerLeft= 0 PointerRight= 4
PointerMid= 2
Recsiv= 2 目前Lst= [50, 60]
Enter Recursive Again!!
Recsiv= 3 Lst= [50, 60, 45, 30, 90, 20, 80, 15]
PointerLeft= 0 PointerRight= 2
PointerMid= 1
Recsiv= 3 目前Lst= [50]
Enter Recursive Again!!
Recsiv= 4 Lst= [50, 60, 45, 30, 90, 20, 80, 15]
PointerLeft= 0 PointerRight= 1
PointerMid= 0
Recsiv= 4 目前Lst= []
Enter Recursive Again!!
Recsiv= 5 Lst= [50, 60, 45, 30, 90, 20, 80, 15]
已不符遞迴條件!!
Recsiv= 5 回上層,進入遞迴前的堆疊,繼續往下執行,Lst= []
Recsiv= 5 回上層,進入遞迴前的堆疊,繼續往下執行,Lst= [50]
Recsiv= 5 回上層,進入遞迴前的堆疊,繼續往下執行,Lst= [50, 60]
Recsiv= 5 回上層,進入遞迴前的堆疊,繼續往下執行,Lst= [50, 60, 45, 30]
Final Result: [50, 60, 45, 30, 90, 20, 80, 15]
+++++++++++++++++++++++++++++++
import math
def merge(items,p,q,r,LorR):
n1=q-p+1
n2=r-q
L=[]
R=[]
for i in range(0,n1):
L.append(items[p+i])
for j in range(0,n2):
R.append(items[q+j+1])
L.append(math.inf)
print("merge前:L=",L)
R.append(math.inf)
print("merge前:R=",R)
i=0
j=0
for k in range(p,r+1):
if L[i] <=R[j]:
items[k]=L[i]
i=i+1
else:
items[k]=R[j]
j=j+1
print("Recsiv=",Recsiv,"merge結束後,目前items=",items)
print()
Recsiv=0
def mergesort(items,p,r,LorR):
global Recsiv
Recsiv+=1
print("Recsiv=",Recsiv)
print(LorR,"mergesort...","p=",p,"r=",r)
if p<r:
q=math.floor((p+r)/2)
print("p=",p,"q=",q,"r=",r)
print("00LL=",items[p:q+1])
print("00RR=",items[q+1:r+1])
print()
mergesort(items,p,q,"左")
mergesort(items,q+1,r,"右")
print("開始merge...")
merge(items,p,q,r,LorR)
else:
print("已不符遞迴條件!")
print()
items=[5,2,9,7]
#items=[5,2,4,7,1,3,2,6]
mergesort(items,0,len(items)-1,"未分左右")
print(items)
=========
Recsiv= 1
未分左右 mergesort... p= 0 r= 3
p= 0 q= 1 r= 3
00LL= [5, 2]
00RR= [9, 7]
Recsiv= 2
左 mergesort... p= 0 r= 1
p= 0 q= 0 r= 1
00LL= [5]
00RR= [2]
Recsiv= 3
左 mergesort... p= 0 r= 0
已不符遞迴條件!
Recsiv= 4
右 mergesort... p= 1 r= 1
已不符遞迴條件!
開始merge...
merge前:L= [5, inf]
merge前:R= [2, inf]
Recsiv= 4 merge結束後,目前items= [2, 5, 9, 7]
Recsiv= 5
右 mergesort... p= 2 r= 3
p= 2 q= 2 r= 3
00LL= [9]
00RR= [7]
Recsiv= 6
左 mergesort... p= 2 r= 2
已不符遞迴條件!
Recsiv= 7
右 mergesort... p= 3 r= 3
已不符遞迴條件!
開始merge...
merge前:L= [9, inf]
merge前:R= [7, inf]
Recsiv= 7 merge結束後,目前items= [2, 5, 7, 9]
開始merge...
merge前:L= [2, 5, inf]
merge前:R= [7, 9, inf]
Recsiv= 7 merge結束後,目前items= [2, 5, 7, 9]
[2, 5, 7, 9]
Permutation
*******************************************
RecSiv=0
def perm(Lst):
global RecSiv
RecSiv+=1
if len(Lst)<=1:
return Lst
Key=Lst[0]
print("RecSiv=",RecSiv," Key=",Key)
print()
print(Lst[1:],"進入遞迴...")
print()
Remaining=perm(Lst[1:])
print("結束RecSiv=",RecSiv," 後,Remaining=",Remaining)
print()
Result=[]
for Remaining_tmp in Remaining:
for i in range(len(Remaining_tmp)+1):
print("寫入Result前, Remaining_tmp=",Remaining_tmp,"Key=",Key)
Result.append(Remaining_tmp[:i]+Key+Remaining_tmp[i:])
print("目前Result=",Result)
print()
return Result
data="ABC"
print("\n最後結果:",perm(data))
=========
RecSiv= 1 Key= A
BC 進入遞迴...
RecSiv= 2 Key= B
C 進入遞迴...
結束RecSiv= 3 後,Remaining= C
寫入Result前, Remaining_tmp= C Key= B
目前Result= ['BC']
寫入Result前, Remaining_tmp= C Key= B
目前Result= ['BC', 'CB']
結束RecSiv= 3 後,Remaining= ['BC', 'CB']
寫入Result前, Remaining_tmp= BC Key= A
目前Result= ['ABC']
寫入Result前, Remaining_tmp= BC Key= A
目前Result= ['ABC', 'BAC']
寫入Result前, Remaining_tmp= BC Key= A
目前Result= ['ABC', 'BAC', 'BCA']
寫入Result前, Remaining_tmp= CB Key= A
目前Result= ['ABC', 'BAC', 'BCA', 'ACB']
寫入Result前, Remaining_tmp= CB Key= A
目前Result= ['ABC', 'BAC', 'BCA', 'ACB', 'CAB']
寫入Result前, Remaining_tmp= CB Key= A
目前Result= ['ABC', 'BAC', 'BCA', 'ACB', 'CAB', 'CBA']
最後結果: ['ABC', 'BAC', 'BCA', 'ACB', 'CAB', 'CBA']
++++++++++++++++
Key="B"
tmp=["C"]
Result=[]
for i in tmp:
for j in range(len(tmp)+1):
print("j=",j," i[:j]=",i[:j]," Key=",Key," i[j:]=",i[j:])
Result.append(i[:j]+Key+i[j:])
print("Result=",Result)
print()
=========
j= 0 i[:j]= Key= B i[j:]= C
Result= ['BC']
j= 1 i[:j]= C Key= B i[j:]=
Result= ['BC', 'CB']
++++++++++++++++
Key="A"
tmp=["BC","CB"]
Result=[]
for i in tmp:
for j in range(len(tmp)+1):
print("j=",j," i[:j]=",i[:j]," Key=",Key," i[j:]=",i[j:])
Result.append(i[:j]+Key+i[j:])
print("Result=",Result)
print()
=========
j= 0 i[:j]= Key= A i[j:]= BC
Result= ['ABC']
j= 1 i[:j]= B Key= A i[j:]= C
Result= ['ABC', 'BAC']
j= 2 i[:j]= BC Key= A i[j:]=
Result= ['ABC', 'BAC', 'BCA']
j= 0 i[:j]= Key= A i[j:]= CB
Result= ['ABC', 'BAC', 'BCA', 'ACB']
j= 1 i[:j]= C Key= A i[j:]= B
Result= ['ABC', 'BAC', 'BCA', 'ACB', 'CAB']
j= 2 i[:j]= CB Key= A i[j:]=
Result= ['ABC', 'BAC', 'BCA', 'ACB', 'CAB', 'CBA']
Combination
*******************************************
def combine(n,k,Result,index,tmp):
print("n=",n," k=",k," Result=",Result,"index=",index,"tmp=",tmp)
print()
if len(tmp)==k:
Result.append(tmp[:])
print("---len(tmp)==k------------目前 Result=",Result)
print()
return
print("進入For 迴圈前 index=",index,"n+1=",n+1)
#print()
#1,2,3,4 ==>n+1
for i in range(index,n+1):
print("For 迴圈的 i=",i)
tmp.append(i)
print("---tmp.append(i)------------未進入combine前 tmp=",tmp)
#print()
print("---combine(n,k,Result,i+1,tmp)------------再次執行combine......")
#print()
combine(n,k,Result,i+1,tmp)
print("結束 combine 未 pop 前 tmp=",tmp)
tmp.pop()
print("結束 combine 已 pop 後 tmp=",tmp)
print()
return Result
res=combine(4,2,[],1,[])
print("FinalResult=",res)
=========
n= 4 k= 2 Result= [] index= 1 tmp= []
進入For 迴圈前 index= 1 n+1= 5
For 迴圈的 i= 1
---tmp.append(i)------------未進入combine前 tmp= [1]
---combine(n,k,Result,i+1,tmp)------------再次執行combine......
n= 4 k= 2 Result= [] index= 2 tmp= [1]
進入For 迴圈前 index= 2 n+1= 5
For 迴圈的 i= 2
---tmp.append(i)------------未進入combine前 tmp= [1, 2]
---combine(n,k,Result,i+1,tmp)------------再次執行combine......
n= 4 k= 2 Result= [] index= 3 tmp= [1, 2]
---len(tmp)==k------------目前 Result= [[1, 2]]
結束 combine 未 pop 前 tmp= [1, 2]
結束 combine 已 pop 後 tmp= [1]
For 迴圈的 i= 3
---tmp.append(i)------------未進入combine前 tmp= [1, 3]
---combine(n,k,Result,i+1,tmp)------------再次執行combine......
n= 4 k= 2 Result= [[1, 2]] index= 4 tmp= [1, 3]
---len(tmp)==k------------目前 Result= [[1, 2], [1, 3]]
結束 combine 未 pop 前 tmp= [1, 3]
結束 combine 已 pop 後 tmp= [1]
For 迴圈的 i= 4
---tmp.append(i)------------未進入combine前 tmp= [1, 4]
---combine(n,k,Result,i+1,tmp)------------再次執行combine......
n= 4 k= 2 Result= [[1, 2], [1, 3]] index= 5 tmp= [1, 4]
---len(tmp)==k------------目前 Result= [[1, 2], [1, 3], [1, 4]]
結束 combine 未 pop 前 tmp= [1, 4]
結束 combine 已 pop 後 tmp= [1]
結束 combine 未 pop 前 tmp= [1]
結束 combine 已 pop 後 tmp= []
For 迴圈的 i= 2
---tmp.append(i)------------未進入combine前 tmp= [2]
---combine(n,k,Result,i+1,tmp)------------再次執行combine......
n= 4 k= 2 Result= [[1, 2], [1, 3], [1, 4]] index= 3 tmp= [2]
進入For 迴圈前 index= 3 n+1= 5
For 迴圈的 i= 3
---tmp.append(i)------------未進入combine前 tmp= [2, 3]
---combine(n,k,Result,i+1,tmp)------------再次執行combine......
n= 4 k= 2 Result= [[1, 2], [1, 3], [1, 4]] index= 4 tmp= [2, 3]
---len(tmp)==k------------目前 Result= [[1, 2], [1, 3], [1, 4], [2, 3]]
結束 combine 未 pop 前 tmp= [2, 3]
結束 combine 已 pop 後 tmp= [2]
For 迴圈的 i= 4
---tmp.append(i)------------未進入combine前 tmp= [2, 4]
---combine(n,k,Result,i+1,tmp)------------再次執行combine......
n= 4 k= 2 Result= [[1, 2], [1, 3], [1, 4], [2, 3]] index= 5 tmp= [2, 4]
---len(tmp)==k------------目前 Result= [[1, 2], [1, 3], [1, 4], [2, 3], [2, 4]]
結束 combine 未 pop 前 tmp= [2, 4]
結束 combine 已 pop 後 tmp= [2]
結束 combine 未 pop 前 tmp= [2]
結束 combine 已 pop 後 tmp= []
For 迴圈的 i= 3
---tmp.append(i)------------未進入combine前 tmp= [3]
---combine(n,k,Result,i+1,tmp)------------再次執行combine......
n= 4 k= 2 Result= [[1, 2], [1, 3], [1, 4], [2, 3], [2, 4]] index= 4 tmp= [3]
進入For 迴圈前 index= 4 n+1= 5
For 迴圈的 i= 4
---tmp.append(i)------------未進入combine前 tmp= [3, 4]
---combine(n,k,Result,i+1,tmp)------------再次執行combine......
n= 4 k= 2 Result= [[1, 2], [1, 3], [1, 4], [2, 3], [2, 4]] index= 5 tmp= [3, 4]
---len(tmp)==k------------目前 Result= [[1, 2], [1, 3], [1, 4], [2, 3], [2, 4], [3, 4]]
結束 combine 未 pop 前 tmp= [3, 4]
結束 combine 已 pop 後 tmp= [3]
結束 combine 未 pop 前 tmp= [3]
結束 combine 已 pop 後 tmp= []
For 迴圈的 i= 4
---tmp.append(i)------------未進入combine前 tmp= [4]
---combine(n,k,Result,i+1,tmp)------------再次執行combine......
n= 4 k= 2 Result= [[1, 2], [1, 3], [1, 4], [2, 3], [2, 4], [3, 4]] index= 5 tmp= [4]
進入For 迴圈前 index= 5 n+1= 5
結束 combine 未 pop 前 tmp= [4]
結束 combine 已 pop 後 tmp= []
FinalResult= [[1, 2], [1, 3], [1, 4], [2, 3], [2, 4], [3, 4]]
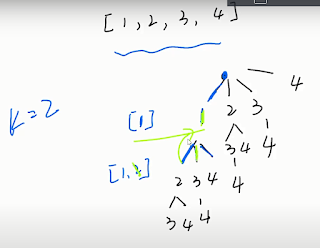
LeetCode 78. Subsets
*******************************************
*******************************************
def BruteForce(Lst):
n=len(Lst)
tmp=[]
maxsum=float("-inf")
for i in range(n):
for j in range(i+1,n+1):
tmp=Lst[i:j]
print(tmp,"sum=",sum(tmp))
maxsum=max(maxsum,sum(tmp))
print("項目 ",Lst[i]," maxsum=",maxsum,"\n")
#print("Final maxsum=",maxsum)
return maxsum
Data=[-2,-3,4,-1,-2,1,5,-3]
Result=BruteForce(Data)
print("FinalResult=",Result)
=========
[-2] sum= -2
[-2, -3] sum= -5
[-2, -3, 4] sum= -1
[-2, -3, 4, -1] sum= -2
[-2, -3, 4, -1, -2] sum= -4
[-2, -3, 4, -1, -2, 1] sum= -3
[-2, -3, 4, -1, -2, 1, 5] sum= 2
[-2, -3, 4, -1, -2, 1, 5, -3] sum= -1
項目 -2 maxsum= 2
[-3] sum= -3
[-3, 4] sum= 1
[-3, 4, -1] sum= 0
[-3, 4, -1, -2] sum= -2
[-3, 4, -1, -2, 1] sum= -1
[-3, 4, -1, -2, 1, 5] sum= 4
[-3, 4, -1, -2, 1, 5, -3] sum= 1
項目 -3 maxsum= 4
[4] sum= 4
[4, -1] sum= 3
[4, -1, -2] sum= 1
[4, -1, -2, 1] sum= 2
[4, -1, -2, 1, 5] sum= 7
[4, -1, -2, 1, 5, -3] sum= 4
項目 4 maxsum= 7
[-1] sum= -1
[-1, -2] sum= -3
[-1, -2, 1] sum= -2
[-1, -2, 1, 5] sum= 3
[-1, -2, 1, 5, -3] sum= 0
項目 -1 maxsum= 7
[-2] sum= -2
[-2, 1] sum= -1
[-2, 1, 5] sum= 4
[-2, 1, 5, -3] sum= 1
項目 -2 maxsum= 7
[1] sum= 1
[1, 5] sum= 6
[1, 5, -3] sum= 3
項目 1 maxsum= 7
[5] sum= 5
[5, -3] sum= 2
項目 5 maxsum= 7
[-3] sum= -3
項目 -3 maxsum= 7
FinalResult= 7
Max Subarray DynamicProgramming
*******************************************
def DynamicProgramming(Lst):
print("原始Lst=",Lst)
n=len(Lst)
for i in range(1,n):
print("目前項目:",Lst[i])
if Lst[i-1]>0:
print("前一項",Lst[i-1],"因為大於0")
Lst[i]=Lst[i]+Lst[i-1]
print("兩項相加,再寫入後 Lst=",Lst,"\n")
ans=max(Lst)
return ans
Data=[-2,-3,4,-1,-2,1,5,-3]
Res=DynamicProgramming(Data)
print("FinalResult=",Res)
=========
原始Lst= [-2, -3, 4, -1, -2, 1, 5, -3]
目前項目: -3
目前項目: 4
目前項目: -1
前一項 4 因為大於0
兩項相加,再寫入後 Lst= [-2, -3, 4, 3, -2, 1, 5, -3]
目前項目: -2
前一項 3 因為大於0
兩項相加,再寫入後 Lst= [-2, -3, 4, 3, 1, 1, 5, -3]
目前項目: 1
前一項 1 因為大於0
兩項相加,再寫入後 Lst= [-2, -3, 4, 3, 1, 2, 5, -3]
目前項目: 5
前一項 2 因為大於0
兩項相加,再寫入後 Lst= [-2, -3, 4, 3, 1, 2, 7, -3]
目前項目: -3
前一項 7 因為大於0
兩項相加,再寫入後 Lst= [-2, -3, 4, 3, 1, 2, 7, 4]
FinalResult= 7
MergeSort (過程參考此例)
*******************************************
def divide(Lst):
if len(Lst)>1:
M=(0+len(Lst))//2
L=Lst[:M]
R=Lst[M:]
print("divide_L=",L)
print("divide_R=",R)
divide(L)
divide(R)
conquer_combine(Lst,L,R)
return Lst
def conquer_combine(Lst,L,R):
L.append(float("inf"))
R.append(float("inf"))
print("conquer_combine前 L=",L)
print("conquer_combine前 R=",R)
i=0;j=0
for k in range(len(Lst)):
if L[i]<=R[j]:
Lst[k]=L[i]
i+=1
else:
Lst[k]=R[j]
j+=1
print("conquer_combine後 Lst=",Lst,"\n")
Data=[-2,-3,4,-1,-2,1,5,-3]
Res=divide(Data)
print("FinalResult=",Res)
=========
divide_L= [-2, -3, 4, -1]
divide_R= [-2, 1, 5, -3]
divide_L= [-2, -3]
divide_R= [4, -1]
divide_L= [-2]
divide_R= [-3]
conquer_combine前 L= [-2, inf]
conquer_combine前 R= [-3, inf]
conquer_combine後 Lst= [-3, -2]
divide_L= [4]
divide_R= [-1]
conquer_combine前 L= [4, inf]
conquer_combine前 R= [-1, inf]
conquer_combine後 Lst= [-1, 4]
conquer_combine前 L= [-3, -2, inf]
conquer_combine前 R= [-1, 4, inf]
conquer_combine後 Lst= [-3, -2, -1, 4]
divide_L= [-2, 1]
divide_R= [5, -3]
divide_L= [-2]
divide_R= [1]
conquer_combine前 L= [-2, inf]
conquer_combine前 R= [1, inf]
conquer_combine後 Lst= [-2, 1]
divide_L= [5]
divide_R= [-3]
conquer_combine前 L= [5, inf]
conquer_combine前 R= [-3, inf]
conquer_combine後 Lst= [-3, 5]
conquer_combine前 L= [-2, 1, inf]
conquer_combine前 R= [-3, 5, inf]
conquer_combine後 Lst= [-3, -2, 1, 5]
conquer_combine前 L= [-3, -2, -1, 4, inf]
conquer_combine前 R= [-3, -2, 1, 5, inf]
conquer_combine後 Lst= [-3, -3, -2, -2, -1, 1, 4, 5]
FinalResult= [-3, -3, -2, -2, -1, 1, 4, 5]
*******************************************
*******************************************